The Impact of Living Abroad on Pension Eligibility and Income
Understanding how pension systems interact becomes crucial when people work across multiple countries throughout their careers. Many countries impose strict eligibility rules that can severely affect retirement income, regardless of the total years worked. Without private savings, individuals...
Sat 2025-03-08
Language: en
Tech Disillusionment
For four decades, I have worked in the tech industry. I started in the 1980s when computing was raw, full of potential, and mainly about solving problems rather than creating new ones. Over the...
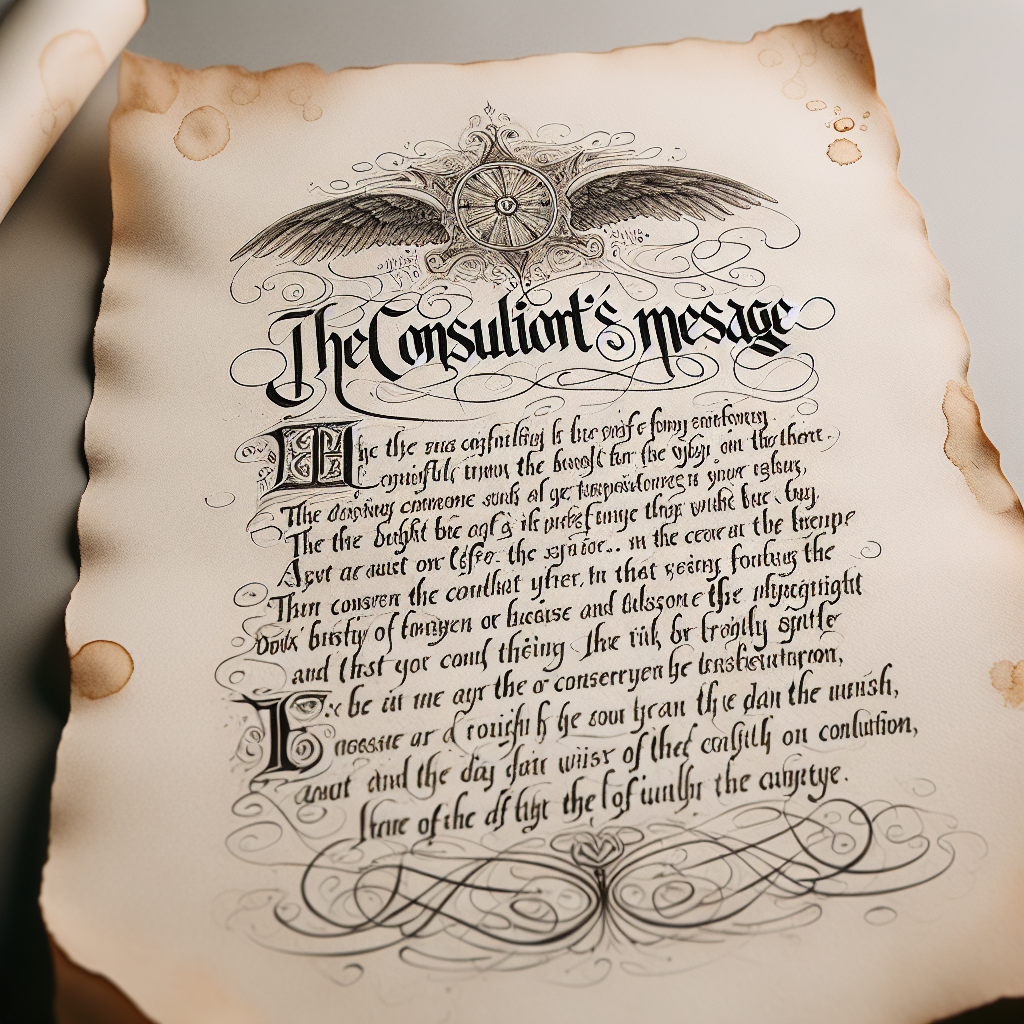
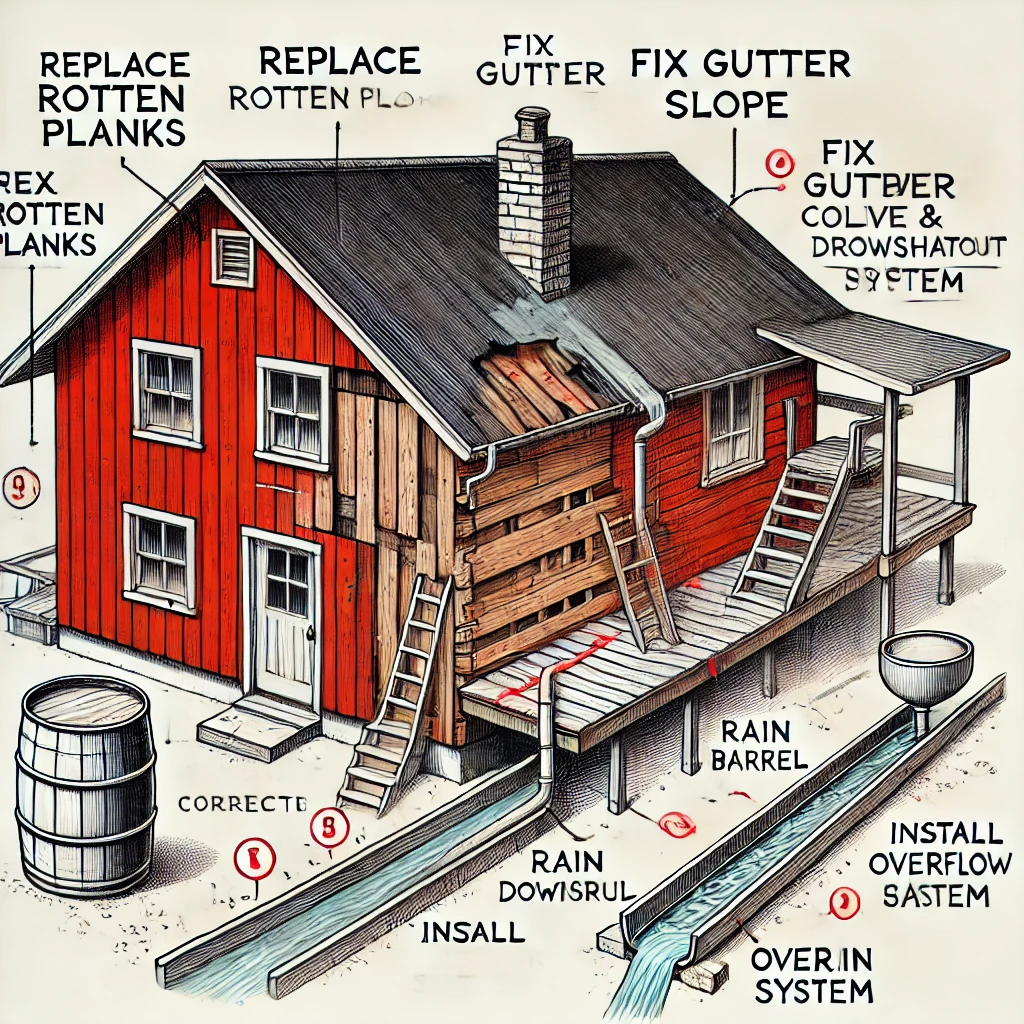
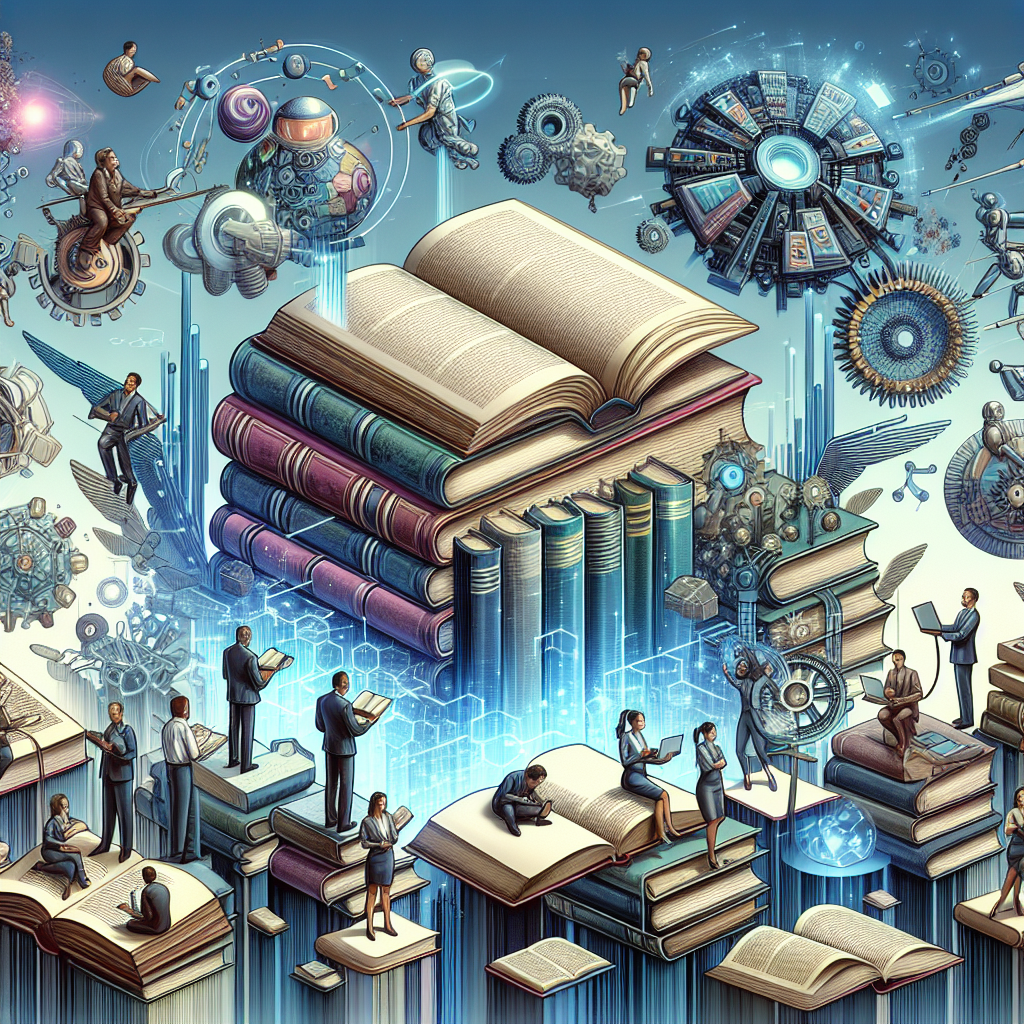
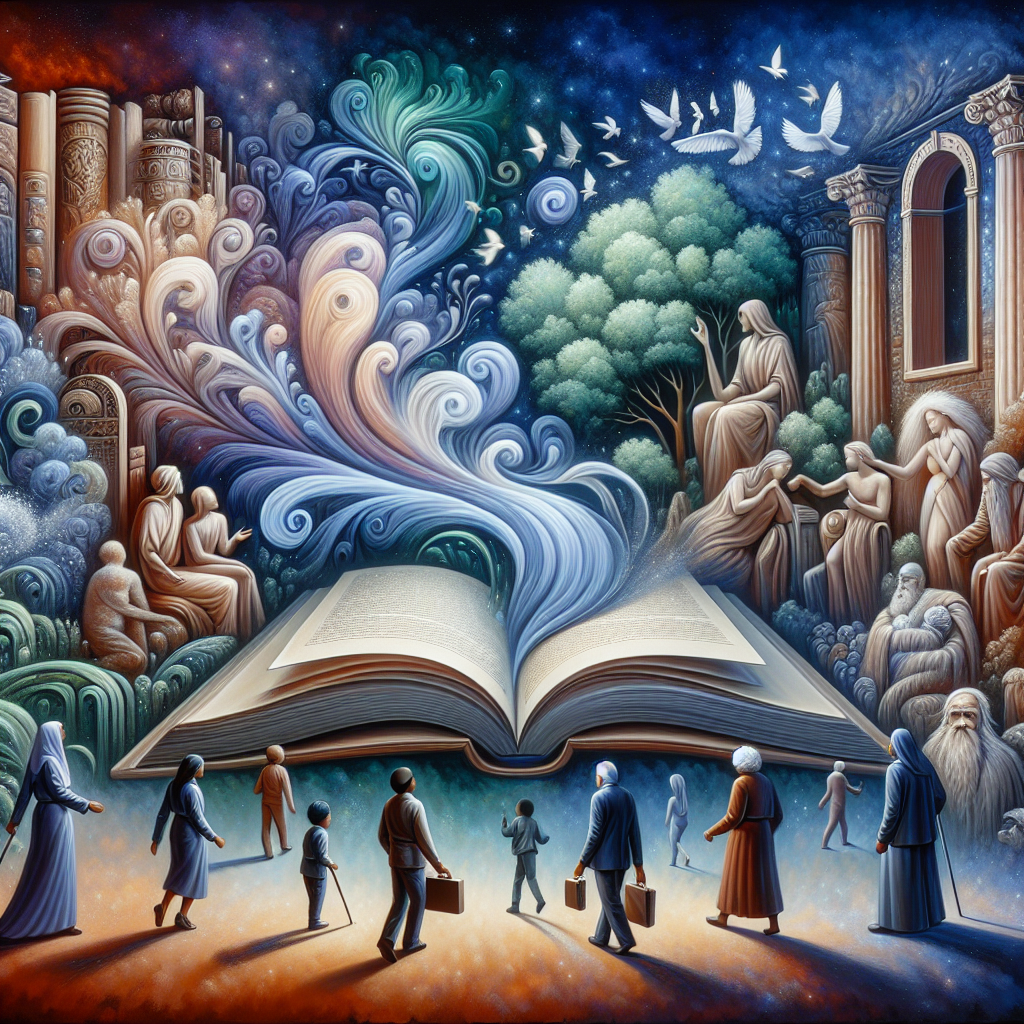
What’s the Environmental Cost of Your Online Activities? From AI Queries to Streaming Video
Scrolling through social media, searching on Google, or chatting with an AI feels like a small, effortless action. But behind every click, there’s a hidden environmental cost. These...
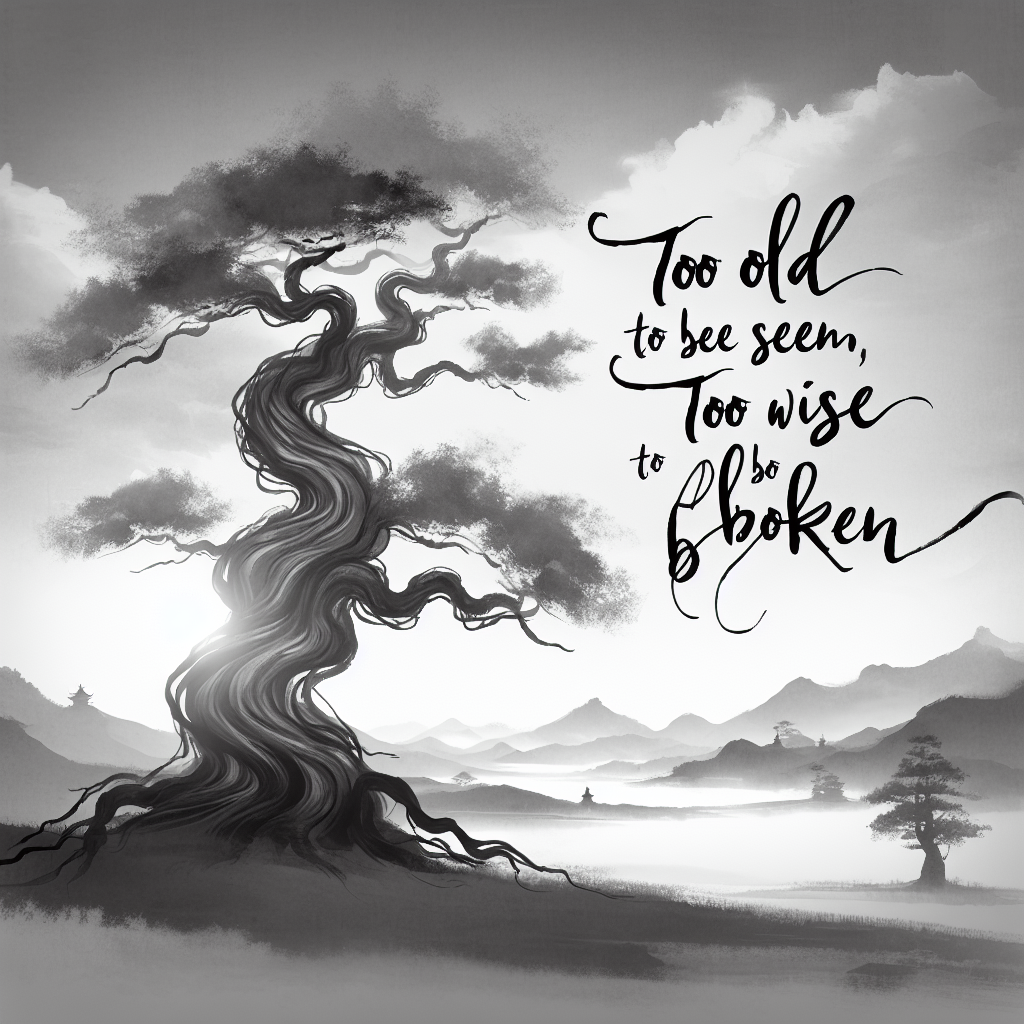
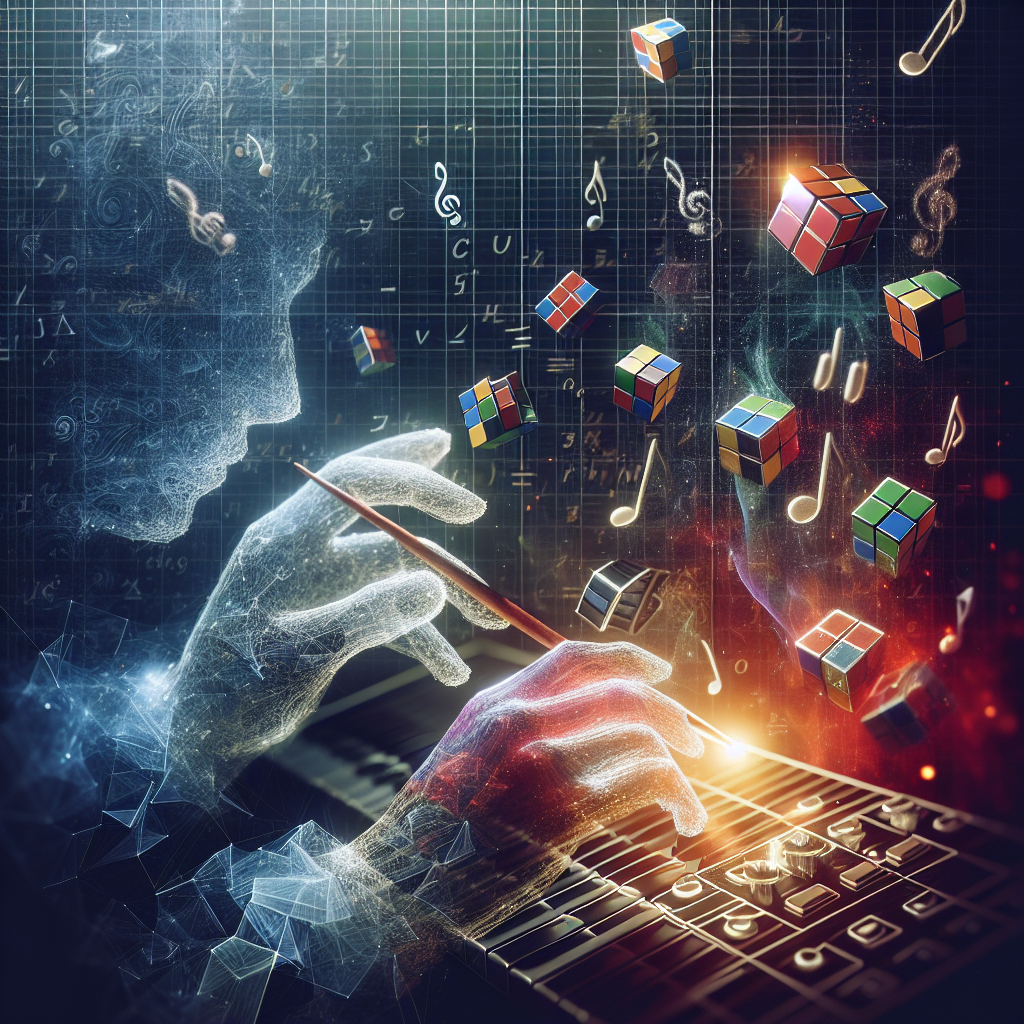
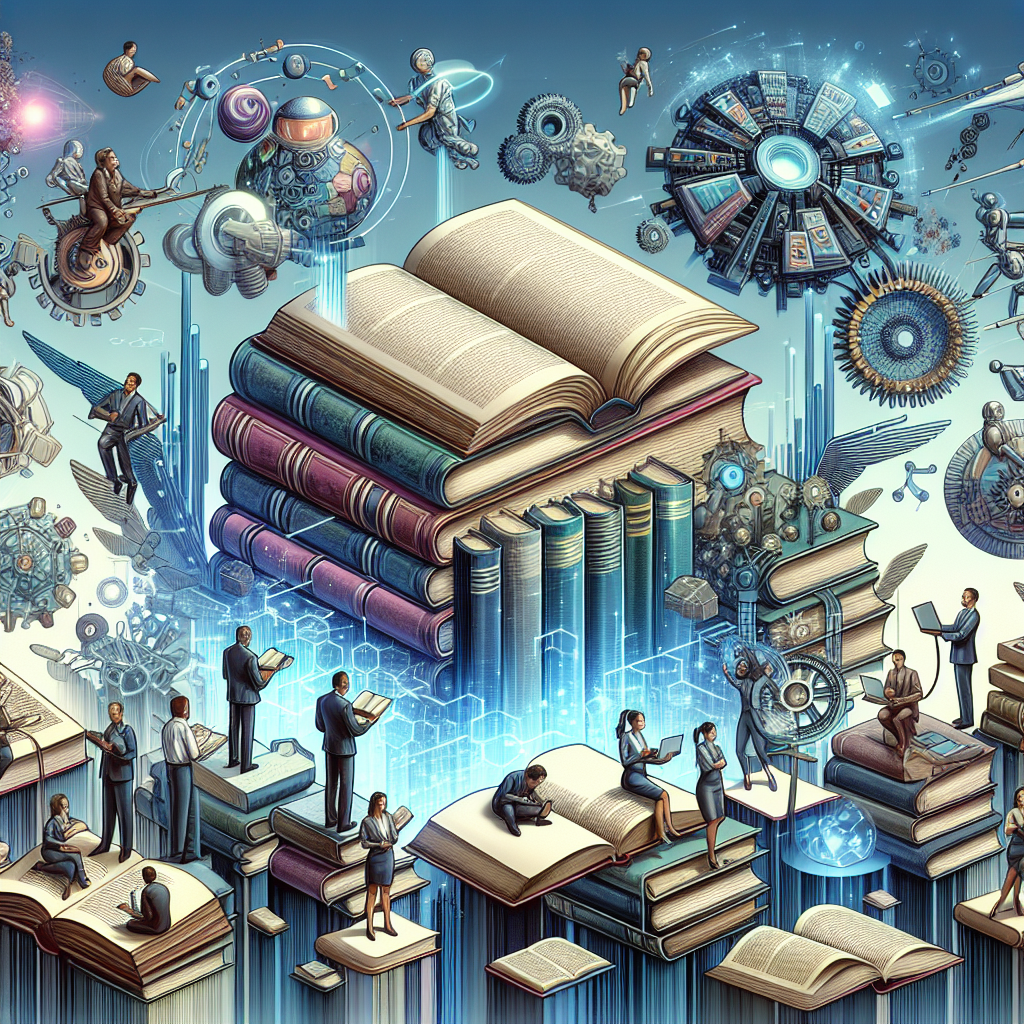
The Role of AI in Enhancing Clear Communication in Multilingual Workplaces
Sun 2025-01-12
Language: en
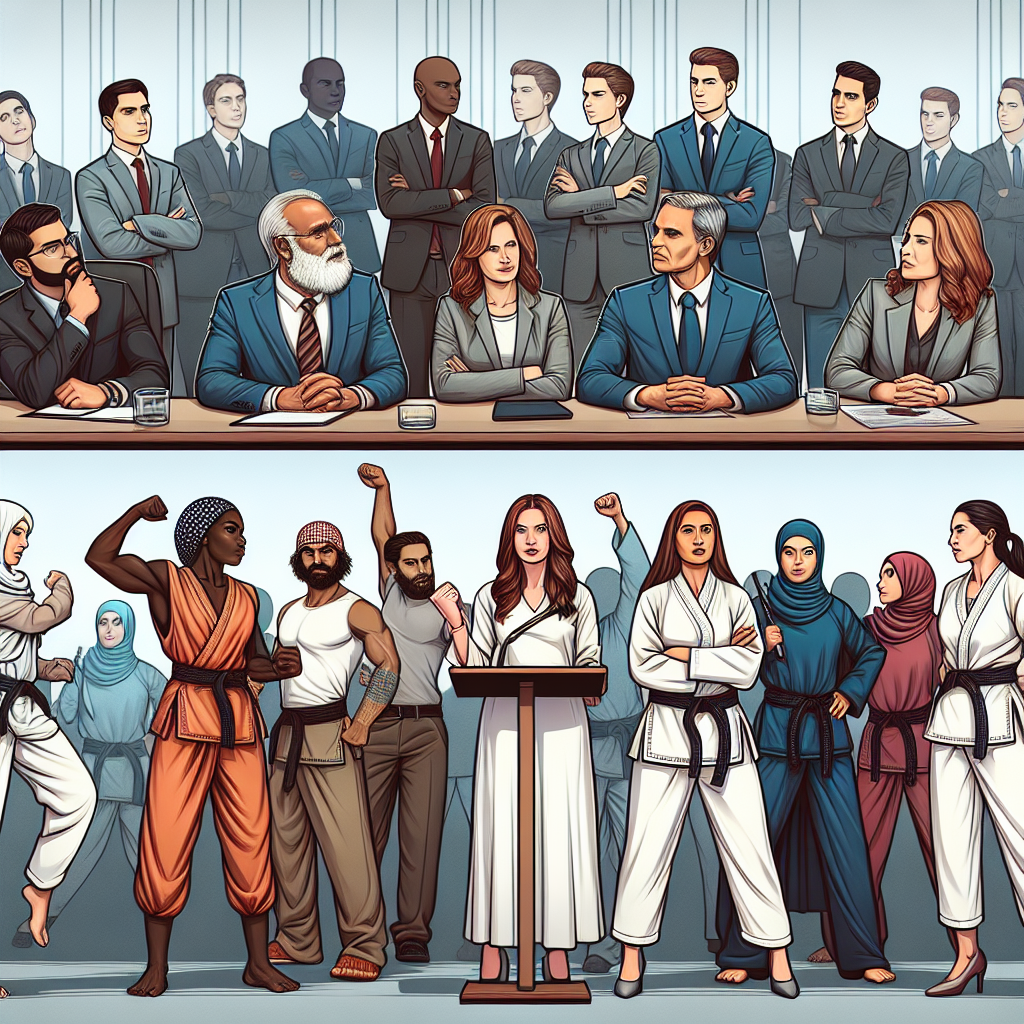
Traits of Highly Knowledgeable Employees That Redefine Workplace Excellence
Mon 2025-01-06
Language: en
The Death of Individuality: Has AI Made Us All the Same?
In a rush to integrate artificial intelligence into our lives, from content creation to decision-making, humanity may have unwittingly created a monster - one that does not destroy us in a...
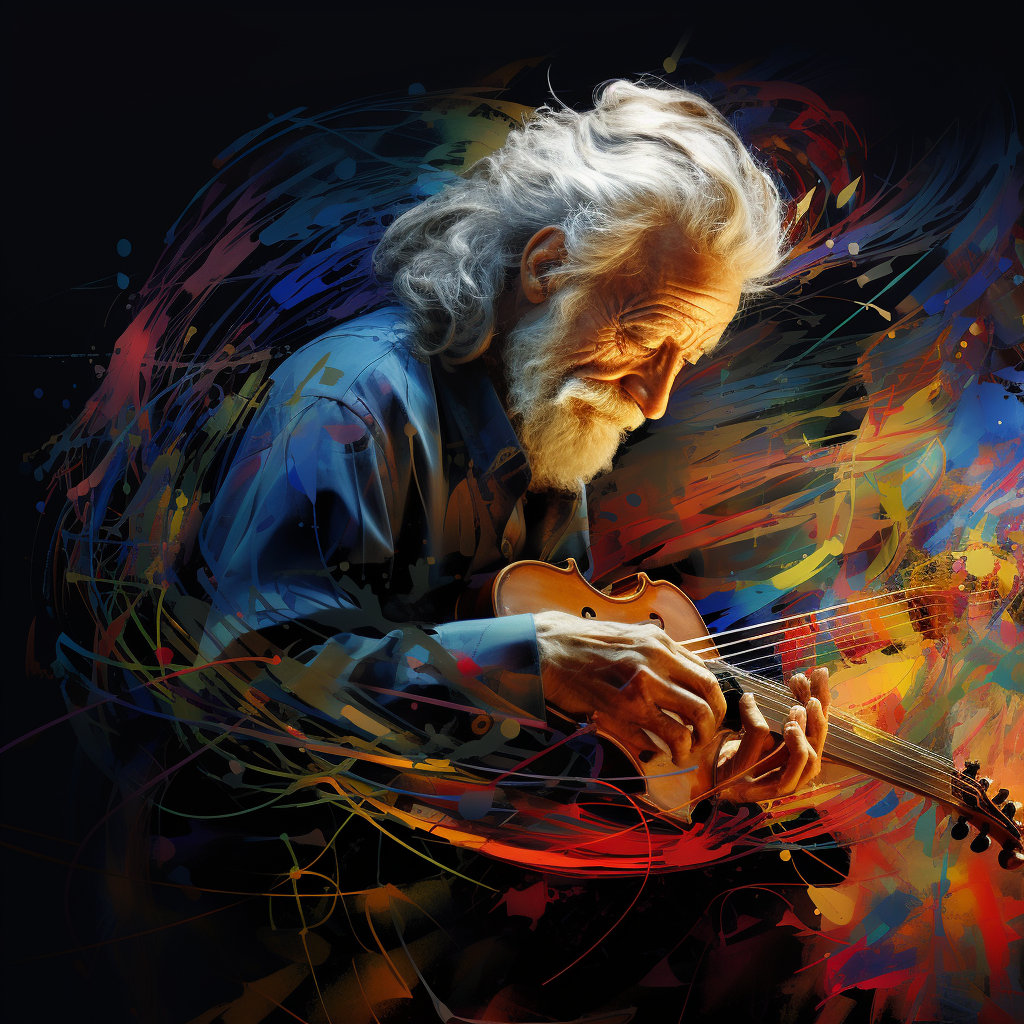
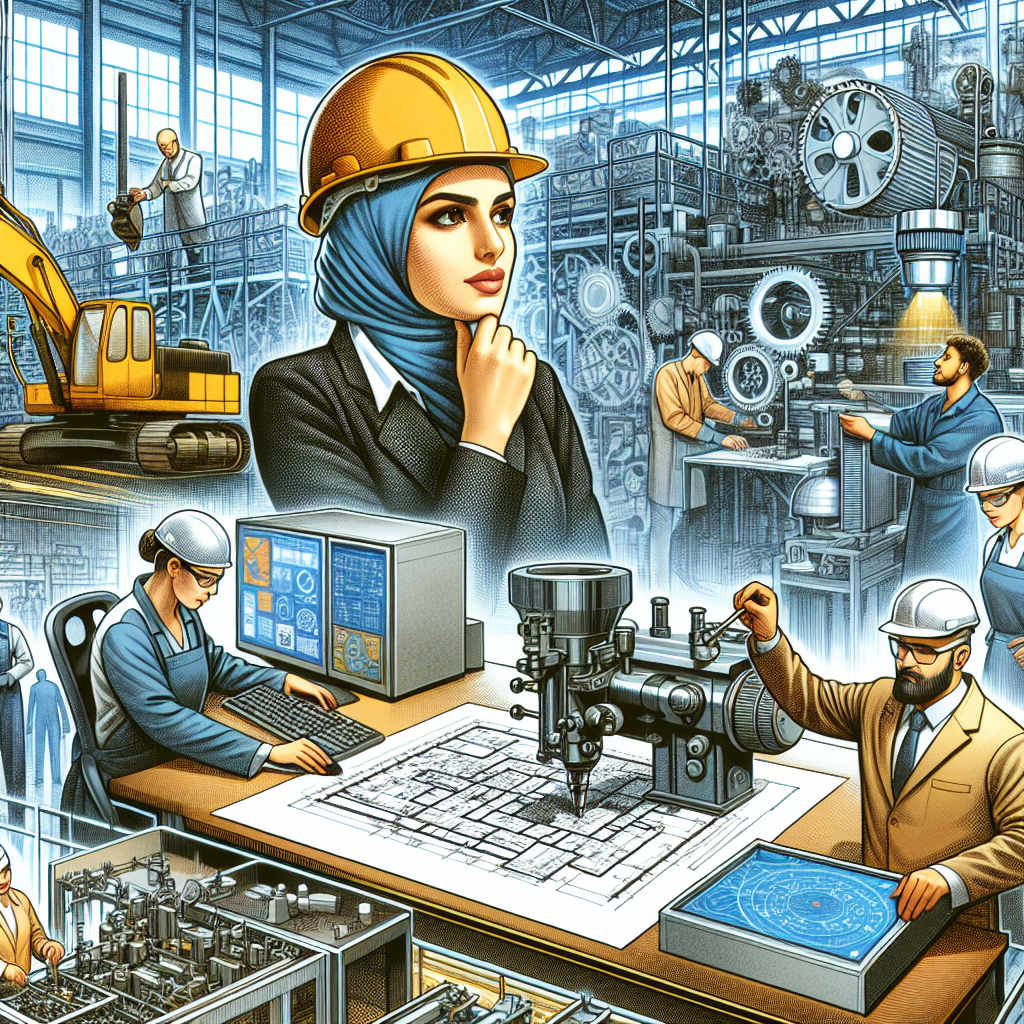
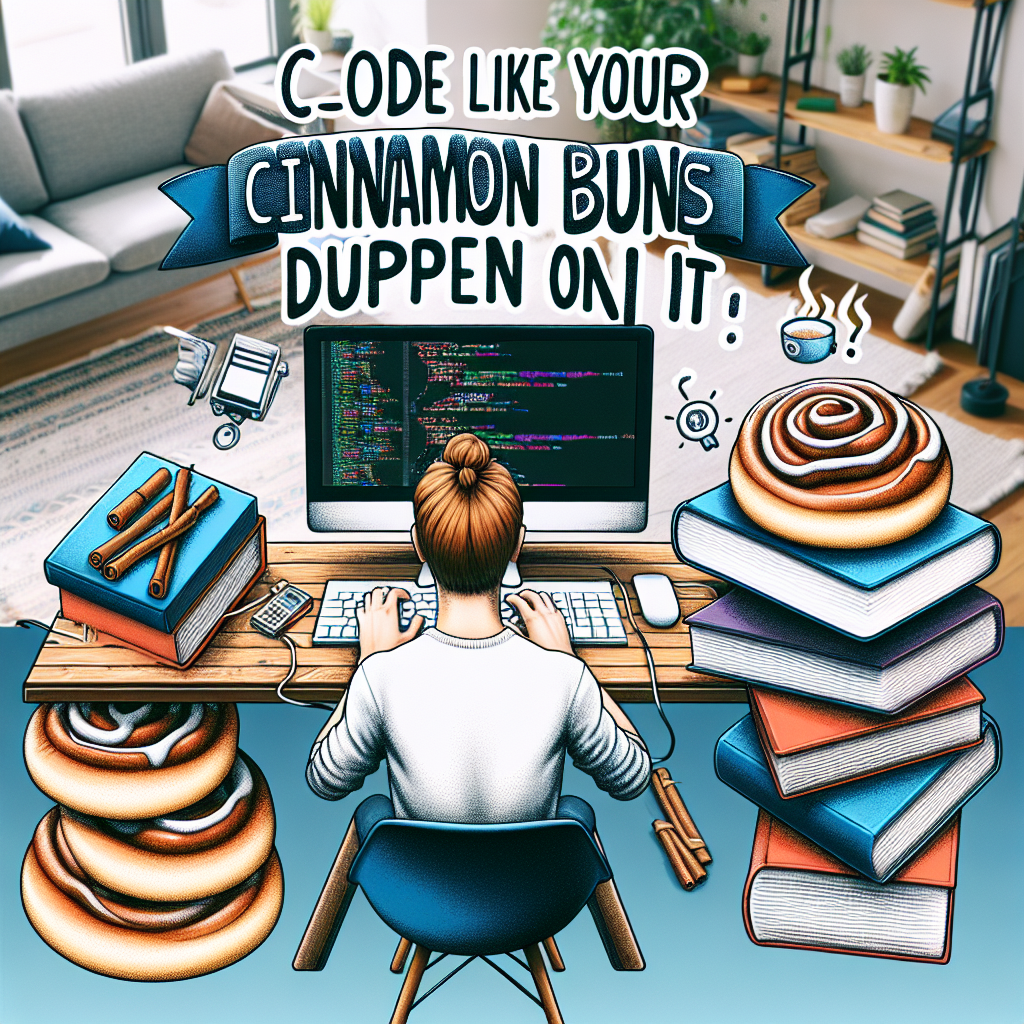
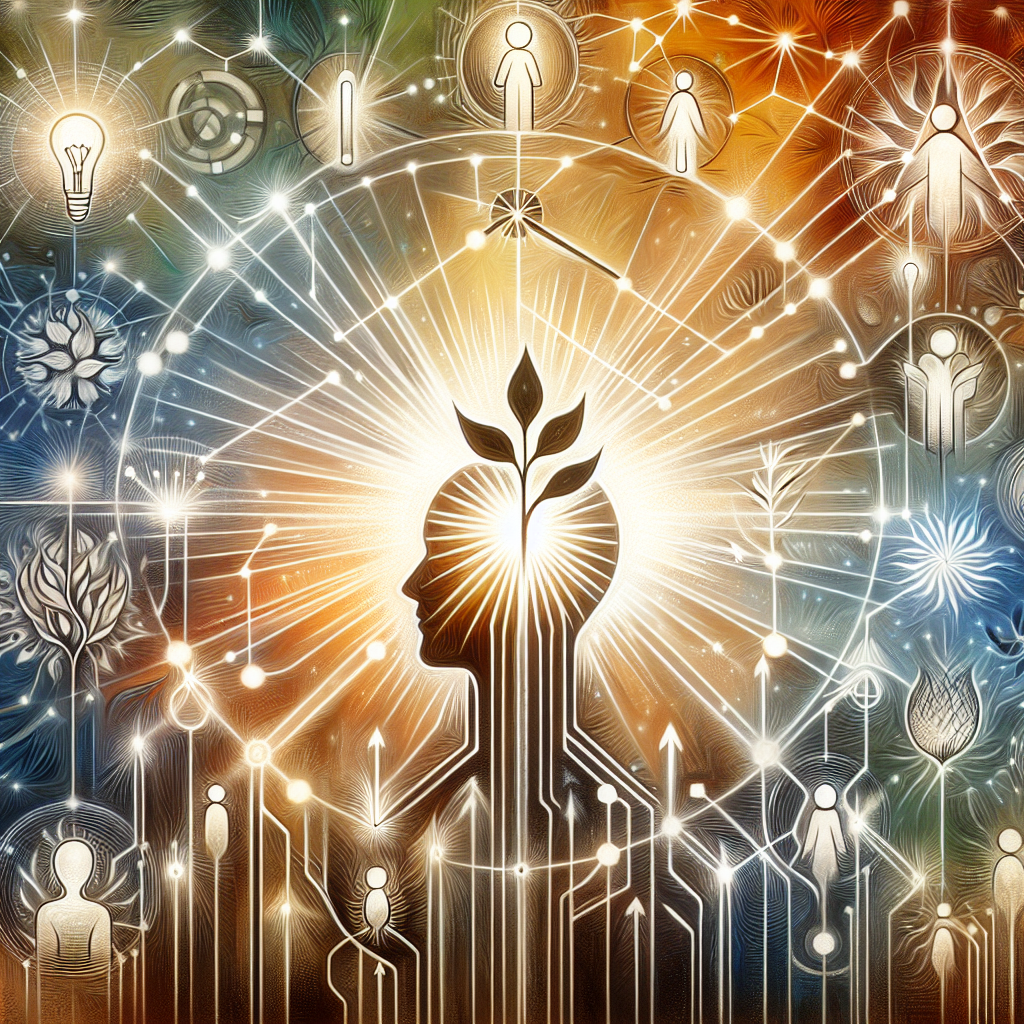