
The ultimate list of tools and libraries for Android development
by bernt & torsten
I recently finished an Android App that uses Google Places API to show cyclists that visit Amsterdam where the nearest bike shop, hotel, atm are while working on this app I did research into Android Libraries and other Android resources, I kept a list of the libraries that I found interesting.
Depending on what you are looking for like a maps library, chart library, or a GUI interface, I have listed what I found below and you can visit the respective links to get a copy or read more. Here is the list of libraries.
Maps
- Google Maps Android API – Instruction on how to use Google Maps Android API
- Google Maps Android Maps Object – Maps are represented in the API by the
GoogleMap
and SupportMapFragment
classes.
Google Places
- Google Places API Web Service – Get data from the same database used by Google Maps and Google+ Local.
Charts
- AChartEngine – Charting Engine.
- EazeGraph – An Android chart and graph library.
- WilliamChart – Android library to create charts.
- MPAndroidChart – A powerful Android chart view/graph view library.
- Pull to refresh – A swipe refresh layout is available in the v4 support library.
- Cardslib – Android Library to build a UI Card.
- AndroidStaggeredGrid – An Android staggered grid view which supports multiple columns with rows of varying sizes.
- AQuery – A light-weight library for doing asynchronous tasks and manipulating UI elements in Android.
- Flow – A small library that helps with describing an app as a collection of moderately independent screens.
- Crouton – Context sensitive notifications for Android.
- DragSortListView – Android ListView with drag and drop reordering.
- MaterialProgressBar – Material design ProgressBar with consistent appearance.
- AndroidFillableLoaders – Android fillable progress view working with SVG paths.
- NexusDialog – Library that allows you to easily and quickly create forms in Android with little code.
Ads Network
- AdMob – AdMob is a mobile advertising company
Backend
- Parse – Build your app on any platform.
- ActionBarSherlock – An extension of the support library designed to facilitate the use of the action bar design pattern across all versions of Android with a single API.
- FadingActionBar – Library implementing a fading effect for the action bar, similar to the one found in the Play Music app
Inputs
- Emojicon – Adds emoticons to your app.
- FloatingLabel – FloatingLabel Allows you to create a blow kind of EditText. Doesn’t have Gradle or Maven Support.
- MaterialEditText – Supporting Floating Labels, Single Line Ellipsis, Max/Min Characters, Helper Text and Error Text with Custom Colors.
- MaterialDrawer – Simple take on a material design navigation drawer.
- PagerSlidingTabStrip – An interactive indicator to navigate between the different pages of a ViewPager.
- Page View indicator – Support for horizontally scrolling ViewPager.
- SlidingMenu – Library to create applications with slide-in menus.
- SlidingTutorial – Simple library that helps to create awesome sliding android app tutorials.
- Android-Transition – Allows the easy creation of view transitions that react to user inputs.
- Android-View-Actions – Makes creating complex animations for views easy.
- Android View Animations – Cute view animation collection.
- NineOldAndroids – Library for using the Honeycomb animation API on all versions of the platform back to 1.0.
- Rebound – Rebound is a java library that models spring dynamics.
- Android-crop – Library project for cropping images.
- Android-Image-Filter – Library project for applying image filters easily.
- CircularImageView – Custom view for circular images while maintaining the best draw performance.
- Fresco – An Android library for managing images and the memory they use.
- Glide – An image loading and caching library for Android focused on smooth scrolling, Recommended by google.
- Picasso – A powerful image downloading and caching library for Android.
- Universal Image Loader – Asynchronous, out of the box loading and caching of images.
- Android-remote-notifications – A Google GCM/Amazon SNS alternative using pull instead of push.
Communication
- Sinch – Use the Sinch APIs to enhance your app with Voice, SMS, Verification, Video, and Instant Messaging.
- DbInspector – Android library for viewing and sharing in-app databases.
- Realm – Realm is a mobile database, a replacement for SQLite & ORMs
- RestorableSQLiteDatabase – A wrapper to replicate android’s SQLiteDatabase with restoring capability.
- ActiveAndroid – Active record style ORM.
- DBFlow – Fast and powerful ORM with compile-time annotation processing.
- NexusData – Object graph and persistence framework for Android.
- ORMLite – Lightweight ORM Java package for JDBC and Android.
- Sugar ORM – Insanely easy way to work with Android Databases.
- Retrofit – Retrofit turns your REST API into a Java interface.
JSON
- Gson – A Java serialization library that can convert Java Objects into JSON and back.
Testing
- AssertJ Android – AssertJ assertions geared towards Android.
- Robotium – Android UI Testing.
- Roboletric – Unit test framework to run tests inside the JVM on your workstation, not in the emulator.
- Mixpanel – Analytics platform to analyze the users.
- MobileAppTracking – Tracking your marketing campaigns across multiple ad networks.
- Byte Buddy – Runtime code generation library with support for Android.
- EventBus – EventBus is a library that simplifies communication between different parts of your application.
- Otto – Event Bus for Android.
- Weak handler – Memory safer implementation of android.os.Handler.
- Bugsnag – Cross-platform error monitoring.
- Crashlytics – Easy crash reporting solution.
- Android Volley – Official Android HTTP library that makes networking easier and faster.
- Asynchronous Http Client – An Asynchronous HTTP Library.
- IceNet – Fast, Simple and Easy Networking for Android
- Ion – Good networking library for Android.
- IceSoap – Easy, asynchronous, annotation-based SOAP for Android.
- OkHttp – An HTTP+SPDY client for Android and Java applications.
- node-android – Run Node.js on Android.
- RoboSpice – the library that makes writing asynchronous network requests easy.
Wireless
- SmartGattLib – SmartGattLib is a Java library that simplifies the work with Bluetooth SMART devices.
Dependency Injection
- AndroidAnnotations – Java annotations with dependency injection.
- Butter Knife – View the “injection” library for Android.
- Dagger – A fast dependency injector for Android and Java.
- RoboGuice – Google Guice on Android,
- Best Practices for Performance – Android Official Training on Performance.
- Developing for Android – Chet Haase’s series, including Memory, Storage, UI Performance.
- Detect and Resolve Performance Problems on Android – Use multiple ways to detect and fix Android Performance Problems.
- Facebook Engineering blogs tagged android – Including many articles on performance.
- Performance Tips – Android’s Official Perf tips.
- Testing Performance of Mobile Apps – Part 1: How Fast Can Angry Birds Run? ##Articles
Game Development
- Libgdx – Cross-platform game engine and SDK. Open Source
- Rajawali – Android OpenGL ES 2.0/3.0 Engine.
- Unity – Cross-platform game engine for mobile.
- Vuforia – Augmented Reality library.
- Android Support library – The Android Support Library package is a set of code libraries that provide backward-compatible versions of the Android framework API.
- Android Priority Job Queue – A Job Queue was specifically written for Android to easily schedule jobs (tasks) that run in the background, improving UX and application stability.
- Android Annotation framework – An Open Source framework that speeds up Android development.
- Caffeine – Speed up your Android development.
- Easy Rating Dialog – A plug-and-play android library for displaying a “rate this app” dialogue.
- Google Play Services – Overview of Google Play Services
- Gradle Retrolambda Plugin – A Gradle plugin for getting java lambda support in Java 6, 7 and Android.
- Guava: Google Core Libraries for Java – Google Core Libraries for Java 6+.
- Hiring a react native Developer – If you’re looking to hire a React Native developer, here are both the hard and soft skills you should be looking for
- RateMeMaybe – Asks the user if (s)he wants to open the Play Store to rate your application when certain requirements are met.
- Tape – A lightning-fast, transactional, file-based FIFO for Android and Java.
- ZXing Android-Integration – Open-source, multi-format 1D/2D barcode image processing library.
- Android Design in Action Video series The video series by Android Design Team of Google.
- Android DevBytes Video Series – It is the technical counterpart of Android Design in Action series.
- Android Hive Tutorials – Very good tutorials for beginners.
- Android Weekly – Newsletter with weekly information about Android.
- Android Asset Studio – Generator for icons and other assets.
- Android Toolbar – A Toolbar for use within application layouts.
- Android UI design resources – It gives you a wide variety of design resources.
- Android-blogs – List with blogs about Android.
- Developing for Android – Article about what you need to consider when developing for Android.
- Device Art Generator – Wraps app screenshots in real device artwork.
- Material Design Palette – A Palette to pick the hex code colour for your material design.
- Pencil Project – An open-source prototyping software.
- Square libraries – Multiple high-quality libraries by square.
Alternative development framework
Android development may not be enough for you, there are other alternatives, especially where cross-platform development can be useful. If you have an app or developing one and want to reach as many devices and platforms, you should consider cross-platform development. There are several options available to you, you can use C# or HTML, CSS and Javascript to build an app, here is some suggestion:
- Anko – Anko is a library that makes Android application development faster and easier.
- Apache Cordova – Cordova-based applications are, at the core, applications written with web technology: HTML, CSS, and JavaScript.
- Corona SDK – Framework to create native iOS and Android Apps (especially Games).
- Groovy on Android – Introduction to Groovy on Android.
- Groovy Language Support for Android – A Gradle plugin to support the Groovy language for building Android apps
- Ionic Framework – A framework to build hybrid apps with mobile-optimized HTML, CSS and JS with AngularJS.
- Macroid – A modular functional UI language for Android.
- NativeScript – An open-source framework to build native iOS and Android apps with JavaScript from a single code base.
- React Native – A framework for building native apps with React by Facebook.
- Reapp.io – Cordova-based framework to build hybrid apps with mobile-optimized HTML, CSS, and JS with ReactJS.
- Scaloid – Library for less painful Android development with Scala.
- SwissKnife – A multi-purpose library containing view injection and threading for Android using annotations.
- Titanium – Open-source framework to create ‘native’ cross-platform apps using JavaScript.
- Xamarin – Framework to create native iOS, Android, Mac and Windows apps in C#.
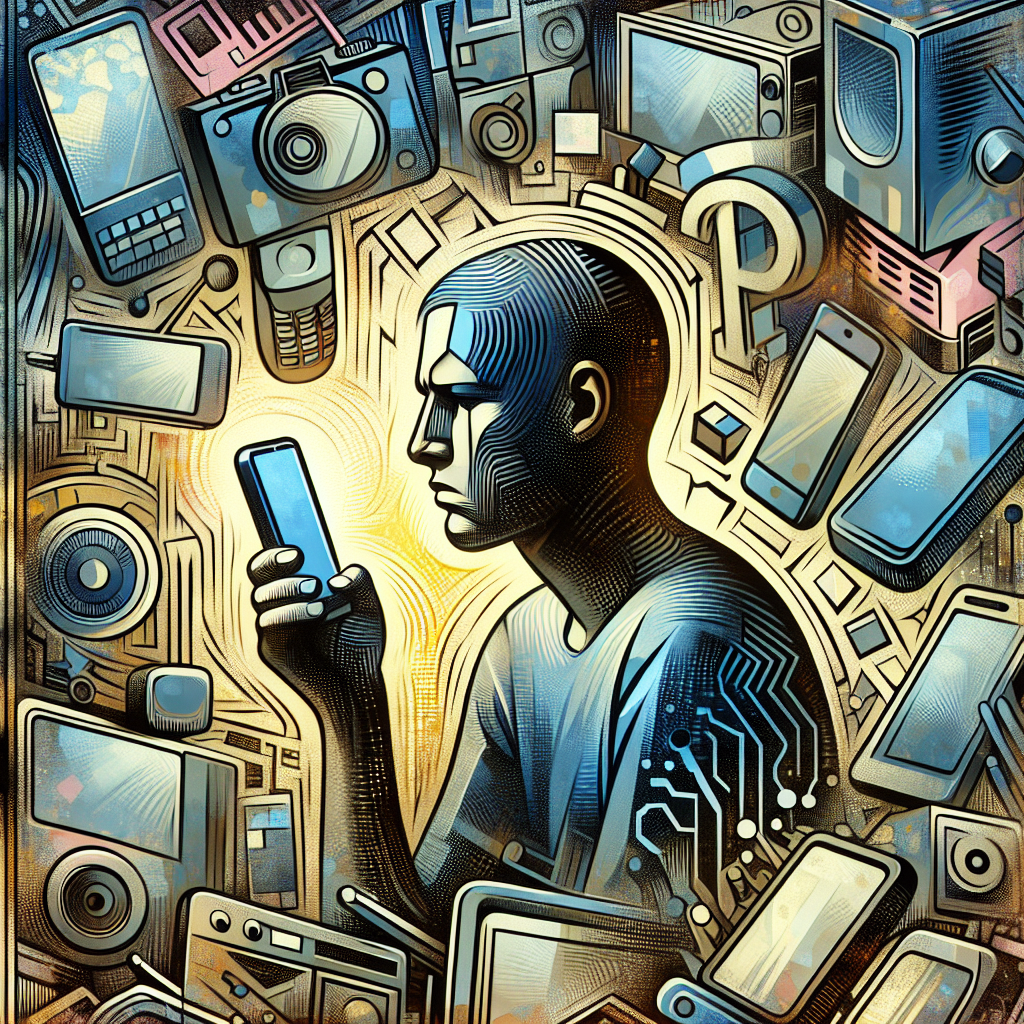
Tech Disillusionment
For four decades, I have worked in the tech industry. I started in the 1980s when computing...
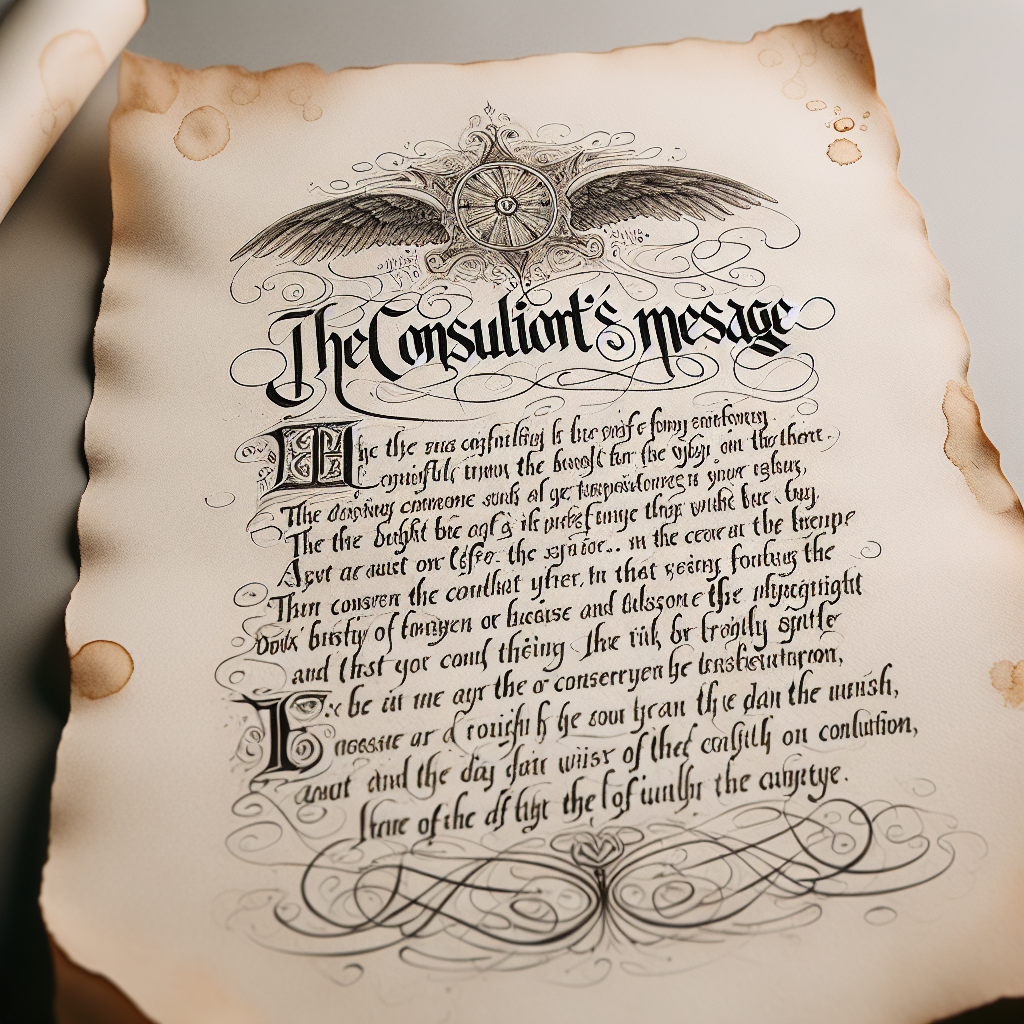
A Poem: The Consultant's Message
On a Friday, cold and gray,
The message came, sharp as steel,
Not from those we...
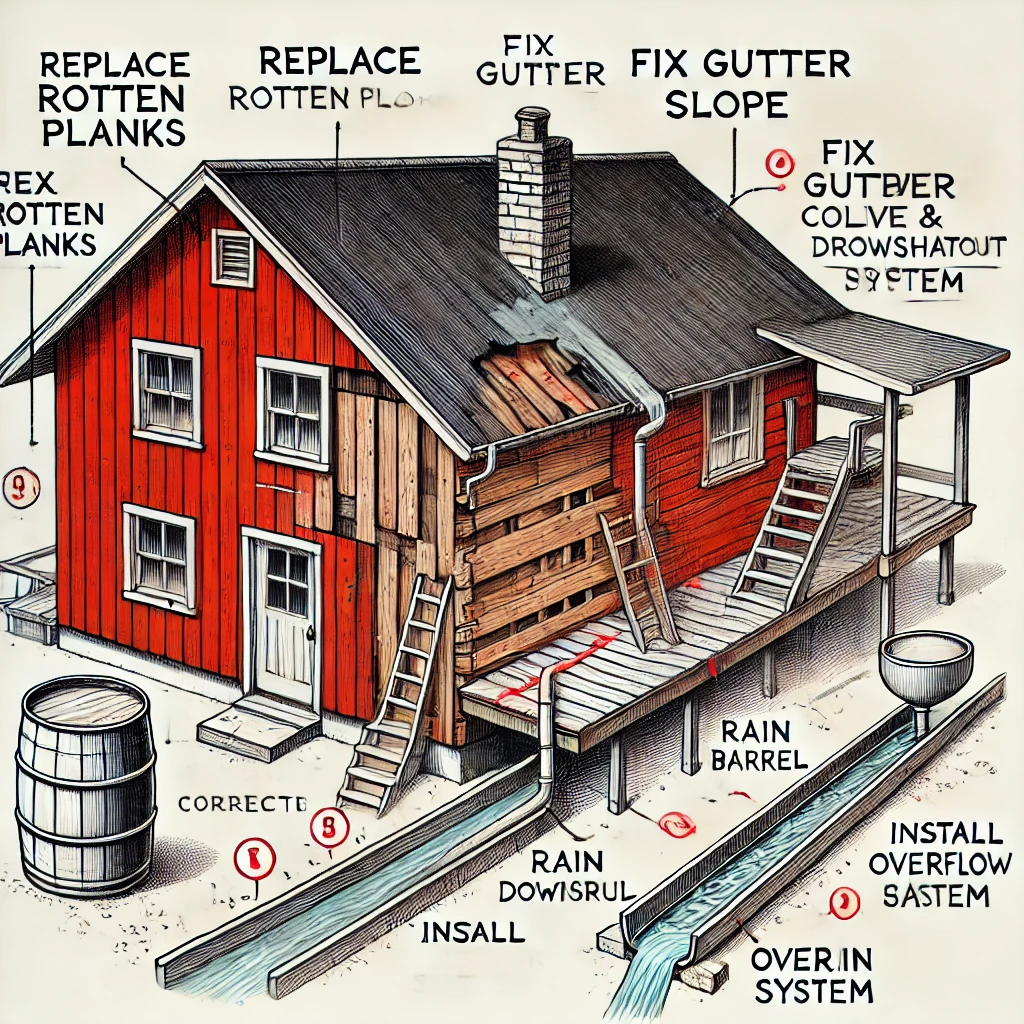
Using AI to Plan Wall Repair and Gutter Installation
In this article, I will share my experience using AI to plan the work required to fix a wall...