
Basic Python RESTful Server bridging PHP with Python
by bernt & torsten
I run into an issue recently, I got a use case to connect to the Luminoso service using their API, Luminoso has an Authentication library in Python, my backend server is written in PHP.
I had to make an API call from my PHP backend to include the Luminoso Python authentication package, so the solution I came up with was to code a basic RESTful Server that would receive a call from my PHP backend, when the request was in the Python code, invoke the Luminoso Python Authentication library to sign an API request to the Luminoso server.
You can get my code from GitHub here Basic-Python RESTful Server
Requirements
Flask micro framework – http://flask.pocoo.org/
Luminoso python client – https://github.com/LuminosoInsight/luminoso-api-client-python
Tested with Python 2.7
If you running CentOS 6.3, read this article Installing python 2.7 on centos 6.3
How to setup
During development, I set up a virtual environment, here is how I did that.
If you are on Mac OS X or Linux, chances are that one of the following two commands will work for you:
$ sudo easy_install virtualenv or even better: $ sudo pip install virtualenv
Once you have virtualenv installed, just fire up a shell and create your own environment. I created a project folder and a env folder within:
$ mkdir myproject $ cd myproject $ virtualenv env
New python executable in env/bin/python
Installing distribute…………done.
Now, whenever you want to work on a project, you only have to activate the corresponding environment. On OS X and Linux, do the following:
$ . env/bin/activate
If you are a Windows user, the following command is for you:
$ env\scripts\activate
If you are using CentOS 6.3
You have to set your Python interpreter.
$ virtualenv -p /usr/bin/python2.7 env
This will use the Python interpreter in /usr/local/bin/python2.7 if you followed the instructions Installing python 2.7 on centos 6.3
To begin using the virtual environment activate:
$ source venv/bin/activate
The name of the current virtual environment will now appear on the left of the prompt (e.g. (env)Your-Computer:your_project UserName$) to let you know that it’s active. From now on, any package that you install using pip will be placed in the env folder, isolated from the global Python installation.
Either way, you should now be using your virtualenv (notice how the prompt of your shell has changed to show the active environment).
Want to learn more about virtual environment – you can find it here.
Now you can just enter the following command to get Flask activated in your virtualenv:
$ pip install Flask
A few seconds later and you are good to go.
As I’m going to use the Luminoso Python client, I need to install it
$ pip install luminoso_api
On Centos 6.3 if you have not changed the names in your virtual environment
$ pip2.7 install Flask $ pip2.7 install luminoso_api
Run the rest-server
$ python rest-server.py &
On Centos 6.3 if you have not changed the names in your virtual environment
$ python2.7 rest-server.py &
You can test it from a browser or a RESTful client
http://127.0.0.1:5000/api/v1.0/test
PHP Code
The web application is built on Codeigniter, here is how I code the call in PHP to call the basic python RESTful server
public function get_luminoso_score($project_name, $article_text) { // Load the rest client spark $this->load->spark('restclient/2.1.0'); // Load the library $this->load->library('rest'); // Set config options (only 'server' is required to work) $config = array('server' => 'http://localhost:5000/'); // Run some setup $this->rest->initialize($config); $this->rest->format('application/json'); $param = array('project_name' => $project_name, 'article_text' => $article_text, 'luminoso_account' => Settings::get('luminoso_account'), 'luminoso_account_name' => Settings::get('luminoso_account_name'), 'luminoso_password' => Settings::get('luminoso_password')); $json = json_decode($this->rest->get('api/v1.0/get_correlation', $param), true); if ($json) { return $json; } else { log_message('error', 'Bad response from Luminoso'); return false; } }
How to adopt for other python clients
It is easy to adopt for other python clients – just replace the Luminoso python client details with the python client that you need to use.

The AI boom – a ticking energy bomb
Yes, the rise of artificial intelligence indeed presents a conundrum, not unlike the many...

Three Ideas To Keep Your Construction Business Running Smoothly
If your construction business is currently struggling, then you need to work out how to get...
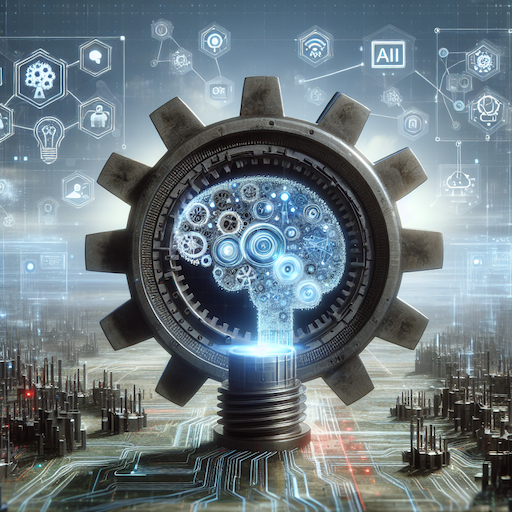
Business vs. GPTs: Navigating the Challenges of Personalized AI
The recent proclamation by OpenAI has caught the attention of $20 ChatGPT Plus subscribers,...